Overview
In this tutorial, we will show you a quick way to receive inbound messages on your SMS-enabled Flowroute number using ngrok and Python's web framework, Flask. ngrok is great for local development when you are not ready to:
- set up a web server
- mess with DNS
- deploy your code to production
In order to test Flowroute's inbound SMS quickly, we will use ngrok to create a tunnel to your localhost, and run an example Flask app that will let you receive an SMS on your Flowroute number.
New to SMS with Flowroute?
If you want to understand the basics of how inbound SMS is handled with Flowroute, check out Receive an Inbound Message.
- An activated Flowoute account
- A Flowroute phone number
- Python 2.6 or newer
- Flask framework
- json module
- ngrok
1. Set up ngrok and establish a secure tunnel
If you haven't downloaded and installed ngrok on your local machine, here's the link again. Open a command shell session and run ngrok on port 8080 to work with our Flask app settings.
ngrok http 8080
Additional ngrok Features
Anyone who can guess your tunnel URL can access your local web server unless you protect it with a password. We will not cover ngrok's auth configuration option which you can enable upon signup. Additionally, if you sign up for a paid ngrok account, you will get access to advanced features such as custom domain and TLS bindings.
2. Set up and run example Flask application
Copy and paste the following Python script into your text editor of choice. Make sure there aren't any extra tabs or spaces to avoid errors.
Example Flask App
from flask import Flask, request
import json
app = Flask(__name__)
app.debug = True
@app.route('/inboundsms', methods=['POST'])
def inboundsms():
json_content = request.json
print json.dumps(json_content)
return "OK"
if __name__ == '__main__':
app.run(
host="0.0.0.0",
port=int("8080")
)
Save the above as inbound_sms.py. Next, open another command shell window or tab and run the file.
python path_to_file/inbound_sms.py
You should now have a Flask-based HTTP server running on local port 8080.
3. Configure inbound SMS for your new ngrok tunnel
Note that we have registered our route as /inboundsms. Copy the forwarding URL from your ngrok session and the app route into the SMS Callback field of your Manage account.
Example SMS Callback
http://5eccb41a.ngrok.io/inboundsms
4. Access ngrok web interface for debugging
ngrok provides a real-time web UI where you can inspect all of the HTTP traffic running over your tunnels. You can access yours by pasting http://127.0.0.1:4040 into a web browser.5. Text your Flowroute phone number
- Select a phone number on the Manage your DIDs tab.
- Send a test SMS from your mobile phone to the Flowroute number you have selected.
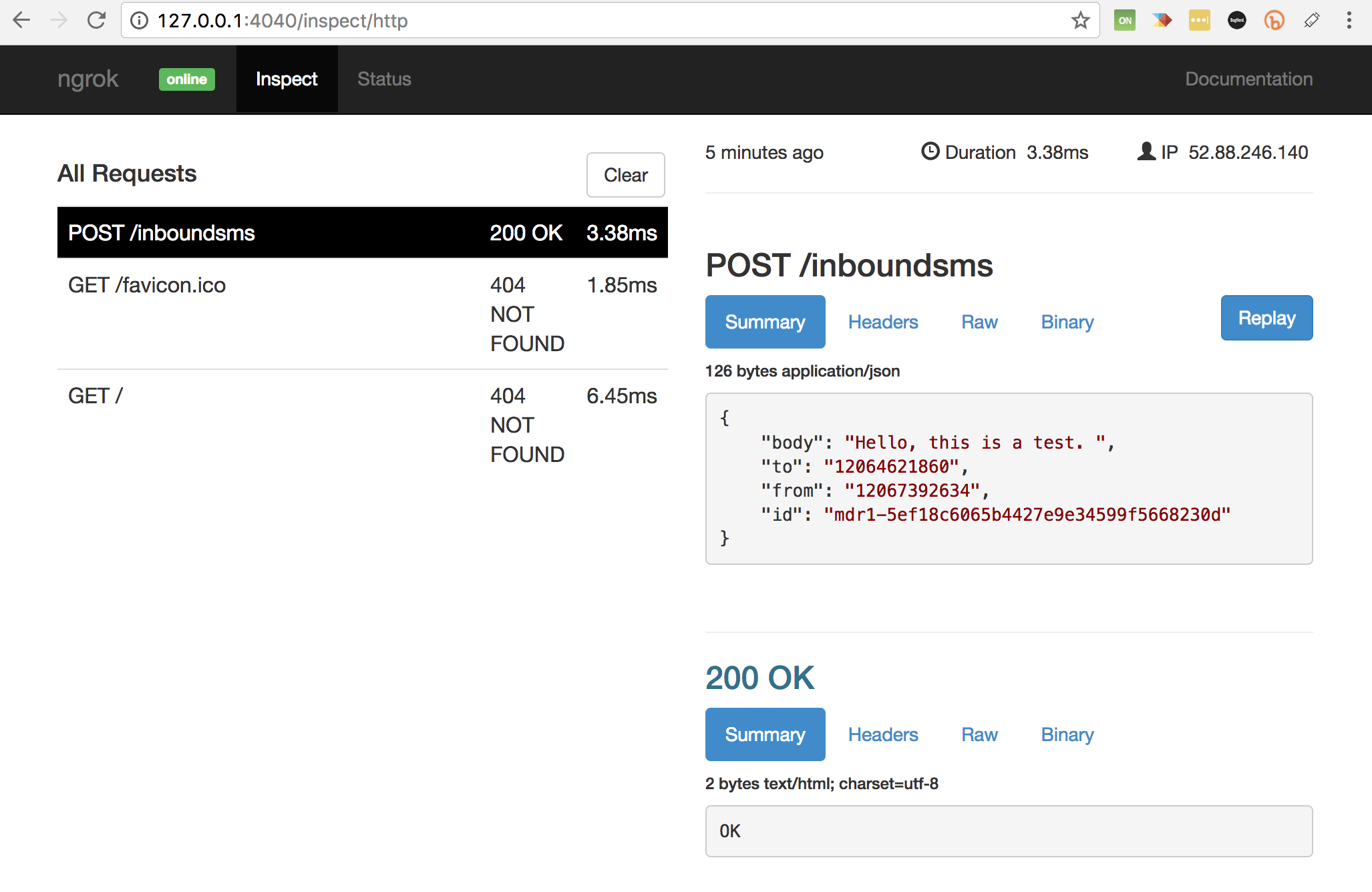
Potential Errors
A typical error that you might encounter is a 404 Not Found. This usually occurs when the SMS Callback isn't set up correctly.
Tips
If the inboundsms endpoint is misbehaving, check that your Manage SMS Callback field has been set to the Forwarding URL followed by / and your defined Flask route. Also, you can click the Replay button on your ngrok web interface to replay the incoming request instead of sending another test message.
Additional Resources
Here are some additional reading materials that might be useful for you.