Overview
AWS (Amazon Web Services) has made a lot of waves in the world of cloud computing with its array of cloud services that you can customize to best fit your IT environment. Flowroute is excited to be leveraging their cloud services through the Serverless Framework. In this tutorial, you will learn how to set up a robust and responsive inbound SMS service. You will be diving into AWS Lambda, Amazon API Gateway, and DynamoDB. Follow along and you’ll be sending text messages to your Flowroute number within 10 minutes or so.
New to SMS with Flowroute?
If you want to understand the basics of how inbound SMS is handled with Flowroute, check out Receive an Inbound Message.
Requirements
- An activated Flowroute account
- A Flowroute phone number
- Node.js v4 or higher (required for Serverless)
- Serverless Framework v1.x
- An AWS account
Setup
1. Add IAM Security Credentials to your local machine
Since the example AWS account in this tutorial features multiple users, we have followed Creating, Modifying, and Viewing Access Keys (Console) to create and retrieve access keys for our specific IAM (Identity and Access Management) user. You can also refer to the official Serverless AWS - Credentials guide. Start a command shell session and create a directory for AWS.
mkdir ~/.aws
After that, create a file for your newly generated access key. You can name it credentials and save it as your default AWS profile.
[default]
aws_access_key_id=<your-key-here>
aws_secret_access_key=<your-secret-key-here>
Alternatively, Serverless provides a convenient way to configure AWS profiles with the help of the serverless config credentials command. Here's an example how you can configure the default AWS profile:
serverless config credentials --provider aws --key ACCESS_KEY --secret SECRET_KEY
2. Install Serverless Framework
The official Serverless documentation covers installation of Node.js and the Serverless Framework in a very straightforward manner. Depending on your npm permissions, you may be required to preface each npm command with sudo.
npm install -g serverless
3. Create Inbound SMS service
Use the create command to create your service. You must also pass in a runtime (e.g., node.js, python etc.) that you would like to write the service in. You will be using python in this guide.
sls create --template aws-python
In running the create command, you will have generated boilerplate template files - handler.py, serverless.yml, and .gitignore. With the aws-python template above, you can define your AWS Lambda Functions, the events that trigger them and any AWS infrastructure resources they require, all in a file called serverless.yml.
4. Create DynamoDB Table
Next, create the DynamoDB table that you will be referencing in serverless.yml and the Python handler file, handler.py. To get started, visit the Create DynamoDB table page. Enter inbound-sms for the Table name, id for Primary Key. If you don’t have any custom settings in mind, leave "Use Default settings" checked.
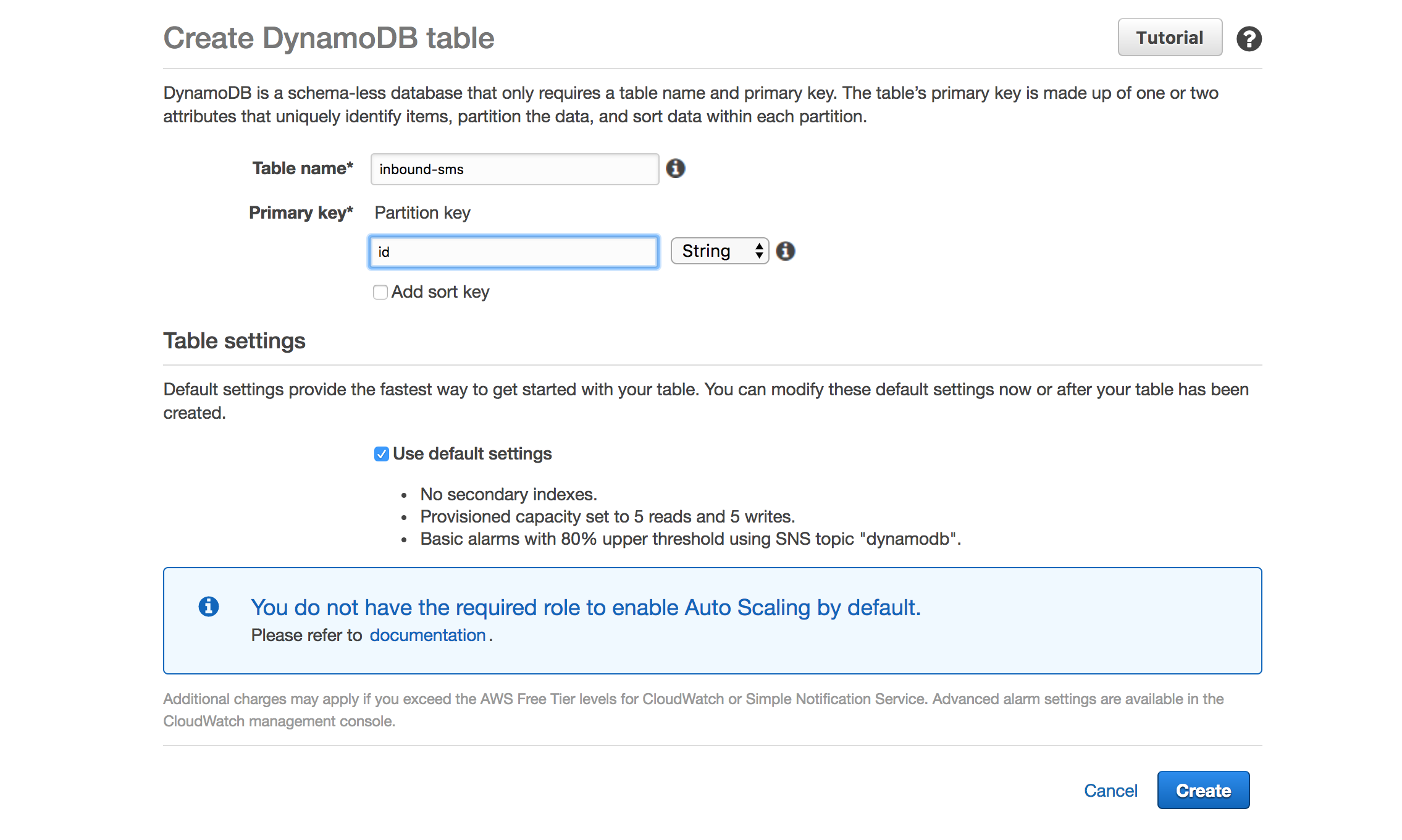
Configure Serverless service
After installing the framework (with its dependencies) and creating the database table, it is time to define your service configuration.
serverless.yml
#Inbound SMS template
frameworkVersion: ">=1.0.0 <2.0.0"
service: receive-inbound-sms
provider:
name: aws
runtime: python2.7
stage: dev
region:
iamRoleStatements:
- Effect: Allow
Action:
- dynamoDB:*
Resource: "arn:aws:dynamodb:::table/inbound-sms" #Amazon Resource name (ARN) of DynamoDB table
functions:
ReceiveInboundSMS:
handler: handler.receive_inbound_sms
events:
- http: POST receive_inbound_sms
Configuration | Description |
---|---|
service | Your Serverless service name; i.e., receive-inbound-sms. |
provider | Information about your service provider for deployment including the Resource required by your function, which in this case is a DynamoDB table. You can find the Amazon Resource Name (ARN) in the Overview tab of your DynamoDB table. | functions | Information about your local function, ReceiveInboundSMS, that will be deployed, its handler, and the events that trigger your function, an HTTP POST request. |
Every serverless.yml translates to a single AWS CloudFormation template and a CloudFormation stack is created from that resulting CloudFormation template. An AWS CloudFormation template describes all the AWS resources that you want (like Amazon EC2 instances or Amazon RDS DB instances) and AWS CloudFormation takes care of provisioning and configuring those resources for you. Other major players have released their own application resource management products - Google now has Cloud Deployment Manager in beta while Microsoft has the Azure Resource Manager.
Define your Lambda Function
Now it’s time to write the Lambda function for your service. Import the json module and boto3, the AWS SDK for Python 3 that lets you specify what resource to use.
handler.py
import json
import boto3
dynamodb = boto3.resource('dynamodb', region_name='us-west-2')
sms_table = dynamodb.Table('inbound-sms') #The DynamoDB table you will be creating in the AWS console
def make_response(code, message, detail=None):
return {
"statusCode": code,
"message": message,
"details": detail
}
def update_dynamo(event):
sms = json.loads(event['body'])
try:
sms_table.put_item(
Item=sms
)
except Exception as e:
return make_response(500, 'Dynamo Error: {}'.format(e), detail=sms)
return make_response(200, "SMS saved to Dynamo", detail=sms)
def receive_inbound_sms(event, context):
response = update_dynamo(event)
return response
Deploy your Serverless service
What's next? Let's take advantage of the Serverless Framework's main benefit, the ability to deploy your newly created service without the hassle of server management! To deploy all of your service, run the following in a command shell window:
sls deploy
For faster deployments, you have the option to only overwrite the zip file of your current function on AWS with the following command:
sls deploy function -f function_name
Use the function name that you have defined in your serverless.yml file as your argument.
Update your SMS Callback URL
Sign into your Flowroute account. Copy and paste the "endpoints" value under Service Information into the SMS Callback field of the API Control tab.
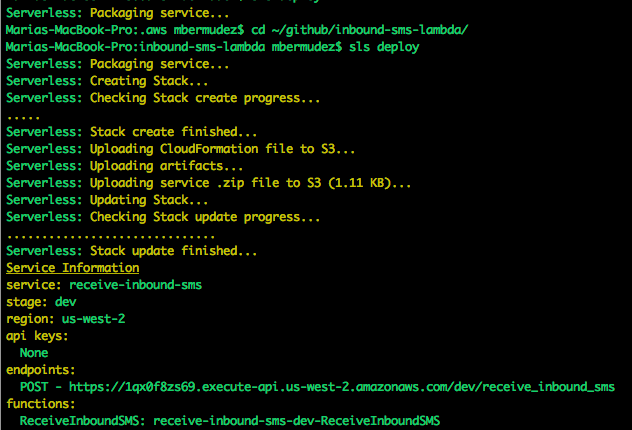
Text your Flowroute phone number
Next, send a test SMS to any of your SMS-enabled Flowroute number. To verify that your message has been sent, visit the Items tab of your DynamoDB table in the AWS console. You should see details of your text message:
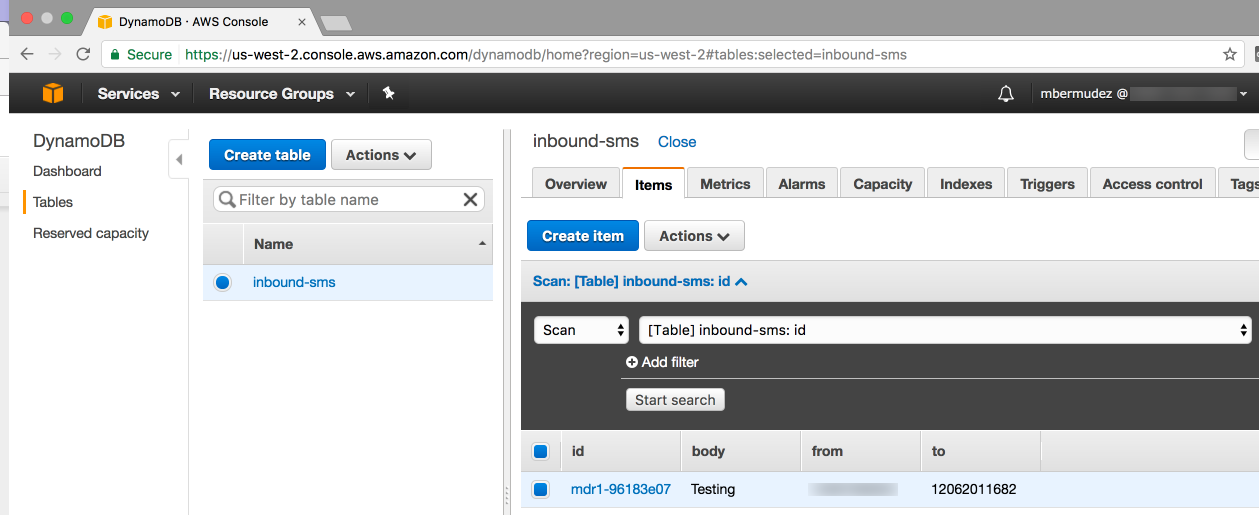
This article barely scratches the surface on the different operations, monitoring tools, and other AWS services you can integrate with DynamoDB. For different ways to access your DynamoDB tables, visit Accessing DynamoDB. To play around with different Serverless Framework - AWS Lambda options, check out this repository of cool Serverless boilerplates and examples.
Related Article
If you're a Python developer working with Flowroute's SMS capabilities, check out our SMS development with ngrok article. Stay tuned for our follow-up quickstart that will help you in your serverless app development in other languages.